More actions
No edit summary |
No edit summary |
||
Line 27: | Line 27: | ||
} | } | ||
</syntaxhighlight> | </syntaxhighlight> | ||
== Extensions == | |||
The challenges were generated using AI (Google's Gemini, but Chat-GPT would have produced something similar). If something looks too challenging, ask AI to explain how you'd go about coding it and see what makes sense! | |||
=== Basic Blinking and Delays === | |||
# The Single Winking Eye: One LED acts as an eye. It stays on (open) for a longer duration, then flashes quickly (winks) for a shorter duration. This practices basic on/off states and different delay values. Vary the "open" and "wink" times. | |||
# Dual Winking Eyes (Asymmetrical): Two LEDs, each acting as an eye, wink independently but with different timings. One eye might wink more frequently than the other. This introduces the concept of controlling multiple outputs concurrently but with individual timing. | |||
# The Blinking Eyelid: One LED is the "eyelid." It starts on (closed), then turns off briefly (opens the eye), and then back on (closes). Experiment with the open time to simulate different eyelid speeds. | |||
=== Introducing Sequences and Patterns === | |||
# The Sequential Gaze: Three LEDs arranged in a row. Turn them on one at a time, creating the illusion of a gaze moving from left to right or vice versa. This introduces the concept of sequential control and using loops. | |||
# The Breathing LED: One LED simulates breathing. It gradually increases in brightness (inhale) and then gradually decreases (exhale). This introduces analog output control (PWM) and smoother transitions. You can do this with a single LED using PWM. | |||
# The Heartbeat: One LED mimics a heartbeat. It pulses with a distinct rhythm, simulating a heartbeat sound. This combines timing with a specific pattern. | |||
=== Adding Complexity=== | |||
# The Reacting Eye: One LED is an eye. It's normally "asleep" (dimly lit). When a button is pressed (or some other simple input), it "wakes up" (becomes brightly lit) for a short period and then returns to the dim state. This introduces basic input and event-driven behavior. | |||
# The Scanning Eyes: Two LEDs represent eyes scanning back and forth. They illuminate one at a time, creating a scanning effect. You can add a third LED in the center that lights up when the "eyes" meet in the middle. | |||
# The Fading Emotion: One LED represents an emotion. It fades in slowly to full brightness (e.g., surprise) and then fades out slowly (e.g., returning to normal). This reinforces PWM and introduces more complex fading patterns. | |||
= Week 2 = | = Week 2 = | ||
= Week 3 = | = Week 3 = | ||
= Week 4 = | = Week 4 = |
Revision as of 16:50, 5 February 2025
This Spring, an adult cohort is exploring animatronics. We'll post helpful resources and our progress along the way here.
Week 1
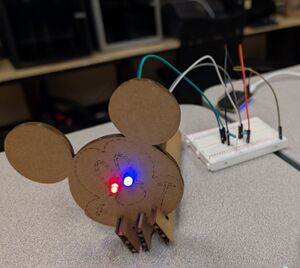
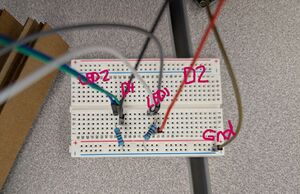
Resources
- Arduino IDE
- Install ESP32 boards in Arduino
- Starter Code
#define BLUE_LED 2 // LED connected to D2 #define RED_LED 4 // LED connected to D4 void setup() { // Initialize the LED pins as outputs pinMode(BLUE_LED, OUTPUT); pinMode(RED_LED, OUTPUT); } void loop() { digitalWrite(BLUE_LED, HIGH); digitalWrite(RED_LED, HIGH); delay(100); digitalWrite(BLUE_LED, LOW); digitalWrite(RED_LED, LOW); delay(100); }
Extensions
The challenges were generated using AI (Google's Gemini, but Chat-GPT would have produced something similar). If something looks too challenging, ask AI to explain how you'd go about coding it and see what makes sense!
Basic Blinking and Delays
- The Single Winking Eye: One LED acts as an eye. It stays on (open) for a longer duration, then flashes quickly (winks) for a shorter duration. This practices basic on/off states and different delay values. Vary the "open" and "wink" times.
- Dual Winking Eyes (Asymmetrical): Two LEDs, each acting as an eye, wink independently but with different timings. One eye might wink more frequently than the other. This introduces the concept of controlling multiple outputs concurrently but with individual timing.
- The Blinking Eyelid: One LED is the "eyelid." It starts on (closed), then turns off briefly (opens the eye), and then back on (closes). Experiment with the open time to simulate different eyelid speeds.
Introducing Sequences and Patterns
- The Sequential Gaze: Three LEDs arranged in a row. Turn them on one at a time, creating the illusion of a gaze moving from left to right or vice versa. This introduces the concept of sequential control and using loops.
- The Breathing LED: One LED simulates breathing. It gradually increases in brightness (inhale) and then gradually decreases (exhale). This introduces analog output control (PWM) and smoother transitions. You can do this with a single LED using PWM.
- The Heartbeat: One LED mimics a heartbeat. It pulses with a distinct rhythm, simulating a heartbeat sound. This combines timing with a specific pattern.
Adding Complexity
- The Reacting Eye: One LED is an eye. It's normally "asleep" (dimly lit). When a button is pressed (or some other simple input), it "wakes up" (becomes brightly lit) for a short period and then returns to the dim state. This introduces basic input and event-driven behavior.
- The Scanning Eyes: Two LEDs represent eyes scanning back and forth. They illuminate one at a time, creating a scanning effect. You can add a third LED in the center that lights up when the "eyes" meet in the middle.
- The Fading Emotion: One LED represents an emotion. It fades in slowly to full brightness (e.g., surprise) and then fades out slowly (e.g., returning to normal). This reinforces PWM and introduces more complex fading patterns.